Ref :How to Build a PDF Viewer with AngularJS & PDF.js | PDFTron
This post will show you how to add a simple PDF viewer to your AngularJS app with PDF.js and the angularjs-pdf
directive. We'll also show you how to match your app's look-and-feel by wrapping it in a custom UI. Like this:
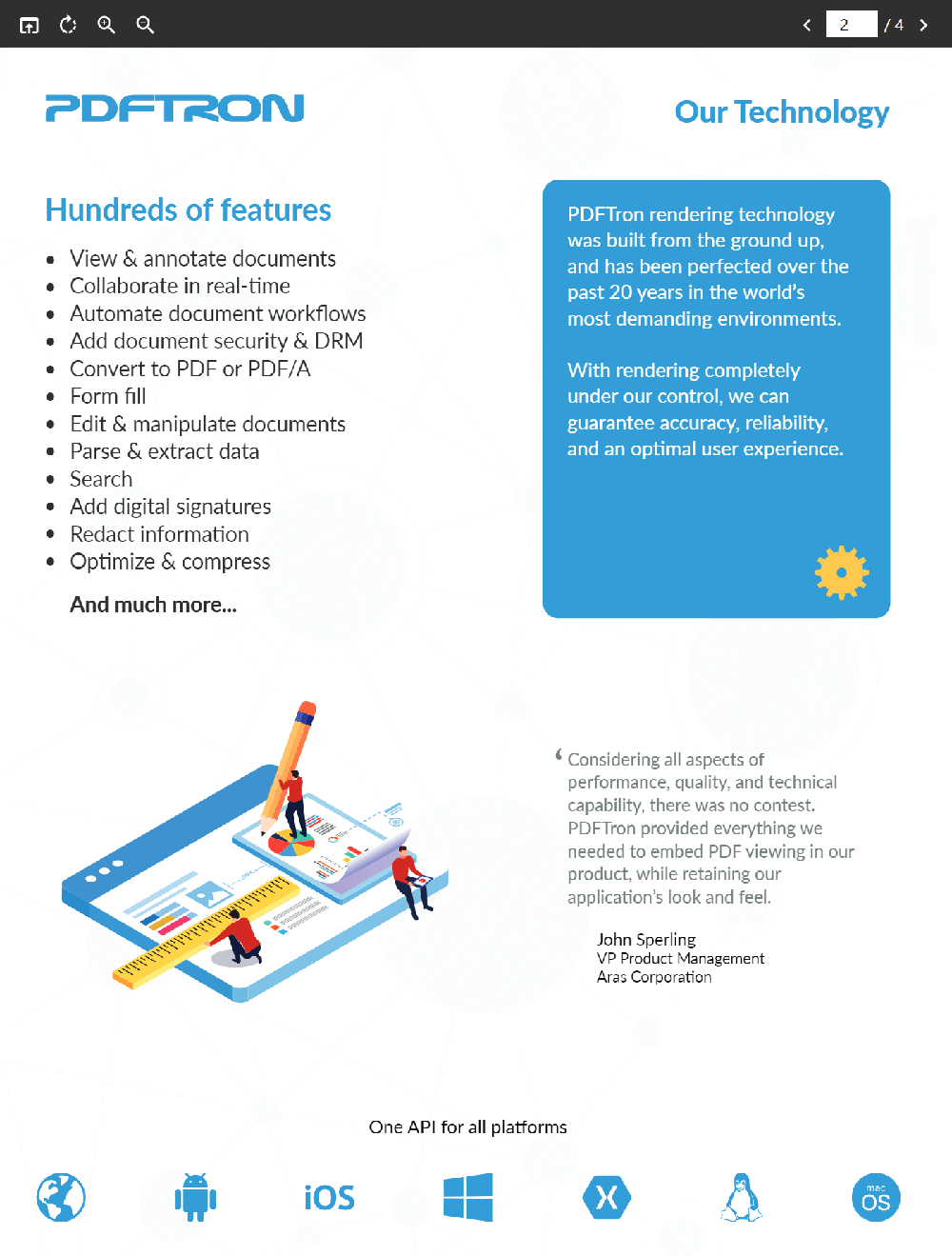
This viewer is ideal for basic viewing use cases, and includes a few basic widgets:
- Opening a local file
- Rotating left
- Zooming in/out
- Previous/next page navigation
The source code for this project is available in our Git repo. We're using PDF.js version 1.6.414
.
Step 1 - Clone the Repo
Once you've created the directory where you want this app to live, clone the repo from the command line:
To change the PDF that's loaded by default, replace PDFTron SDK
and pdftron-sdk.pdf
in docCtrl.js
:
You can now run this basic viewer in your app. Next, we'll customize the UI...
Step 2 - Configuration
The viewer has limited options that can be configured in index.html
:
The scale
value can be page-fit
or a number, the page
value indicates the first page displayed in the viewer, while a usecredentials
value of true
would require a password to open the file (but it does not encrypt the file). See the official README.
The $scope.onProgress
and $scope.onLoad
functions can be used to show an animation while the file is loaded, and then do something when it's finished.
Step 3 - Removing Widgets From The UI
The code for displaying widgets is located in viewer.html
, with widgets wrapped in <span>
tags. To remove (or re-order) the widgets, simply find the tag and adjust as needed.
For example, delete this code to remove the rotation toggle:
Step 4 - Replacing Widget Icons
Widget icons are referenced individually from viewer.html
, so it's easy to swap them out with whatever image you'd like to use:
The above code is for the File Open widget. To use a different image, replace icon/baseline-open_in_browser-24px.svg
with the one you'd like to use.
Step 5 - Changing Widget or Toolbar Styling
Widgets and the toolbar can be styled in style.css
. Here we can adjust the background of the toolbar, widget sizing or padding, and more.
And that's it! You now have a simple AngularJS PDF viewer that matches your app's look-and-feel.
This is a great solution if you just need basic viewing capabilities, and you're not too concerned with PDF.js rendering inconsistencies or failures that can occur (especially the older versions). If you are in the process of evaluating PDF.js be sure to see our guide to evaluating PDF.js.
If you need to add more robust functionality, like annotation, form filling, 100% rendering reliability, and more to your viewer, we offers a JavaScript-based PDF viewer:
- View, annotate, and collaborate in real-time
- Watermark, stamp, form fill, sign, search, and more
- Client-side PDF editing (without a server)
- Document security, DRM, encryption, redaction
- Overprint, ICC color management, color separation
- PDF, MS Office, and 30+ formats
To see it in action check out our WebViewer demo.
Also, it's easy to integrate into your AngularJS project...
Integrating PDFTron WebViewer Into AngularJS Projects
Make sure you have Node.js installed to run the sample.
Start by cloning and installing our WebViewer AngularJS sample project:
And that's it! You've now got a robust and reliable PDF viewer that's easy to customize.
To integrate this into your own app, simply use the directive provided in the sample.
Conclusion
Building a PDF Viewer with AngularJS and PDF.js can be straightforward, but once you start needing more advanced features, 100% reliability, or high-fidelity rendering, open source may not be the best approach.
PDFTron WebViewer has hundreds of features, support for 30+ file formats, a proven rendering engine built right in, and it's simple to integrate with Angular projects.
Check out documentation for our JavaScript PDF library, and get started with your free trial.
If you have any questions about PDFTron's PDF SDK, please feel free to get in touch!
You can find the source code for this blog post at Github.
No comments:
Post a Comment